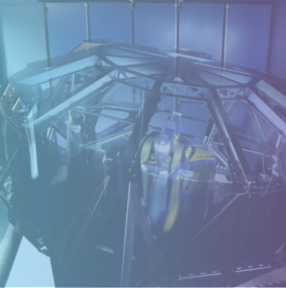
Gulp as a Drush Task Runner
Author: Nick Christensen - Senior Developer
Drupal is great for a number of reasons. Benefits in security, maintenance costs, accessibility, scalability, page optimization and a content type and taxonomy system make it an ideal choice for scalable and resource rich websites. I’d like to focus on specifically one aspect of the page optimizations provided by Drupal. Caching, saves the server a lot of time by saving copies of the current site, rather than doing all of the processing required to build each page for each request.
Drupal caches files quite aggressively in-order to improve page load time. This is awesome for user experience and SEO! Not so awesome when project files are being changed and tested, like they are in development. In order for developers to see the most current version of the site, developers must manually clear their cache! Fortunately Drush provides us with an excellent set of tools for managing the site from the command line. To combat that issue we have come up with a quick gulp script that will keep your development workflow running smoothly.
We wanted a script that would be quick and easy to install and configure on existing sites. What we came up with is a couple npm packages and a short gulpfile with minimal project specific changes. The gulpfile, very simply, watches the theme files for any changes. If a file is changed drush cr
is executed. After drush finishes clearing the cache, gulp will trigger a nice little OS notification.
Lets start with defining our dependencies in our package.json
. gulp-shell is the lynchpin in this situation. With it we can execute bash commands in our gulp tasks! In this case I am using gulp-shell to clear the cache. But first lets take a step back. You will want to run npm install
to insure you have the proper packages.
{ "name": "Sample Project", "version": "0.1.1", "description": "", "main": "gulpfile.js", "dependencies": { "gulp": "^3.9.1", "gulp-load-plugins": "^1.5.0", "gulp-notify": "^3.2.0", "gulp-shell": "^0.6.5" } }
Next lets import our desired modules. Gulp is a give in, but gulp-load-plugins is nifty for when you are working with many other plugins (we aren’t in this case but might as well have an easy scalability option). Basically it takes the gulp dependencies stores in your package.json and attaches them to an object, in our case $
.
var gulp = require('gulp'); var $ = require('gulp-load-plugins')();
After that lets define some minor configuration settings. This is the only section you should need to change between projects. It should be pretty self explanatory. themeDir
is the path to your theme directory. drushPath
is the path to the drush executable stored in your drush installation. In our case we use composer to install drush and it is stored in the vendor directory.
var themeName = 'sample-theme'; var config = { themeDir: './themes/' + themeName, drushPath: '/vendor/drush/drush/drush' };
After that we can define the ‘drushcr’ gulp task, and its process. gulp.src()
takes all the files and directories of the project (current dir and child files / dirs) as a context and pipes it into .pipe()
. Our first action is to execute drush cr
using our config vars and gulp-shell . On success we can notify the developer that the task is complete!
gulp.task('drushcr', function() { return gulp.src('', { read: false }) .pipe($.shell([ './vendor/drush/drush/drush cr', ])) .pipe($.notify({ title: "Caches cleared", message: "Drupal caches cleared.", onLast: true })); });
Thanks to gulp-notify our notifications are even themed to our OS and displayed in the top right corner of your screen by default: (Mac / Ubuntu)
Lastly, we setup the watch hooks using gulp.watch(filepattern, array('taskname'))
. With our config.themeDir
we can identify all of files that we need to react too when changed.
gulp.task('default', ['drushcr'], function() { gulp.watch(config.themeDir + "/sass/**/*.scss", ['drushcr']); gulp.watch(config.themeDir + "/js/**/*.js", ['drushcr']); gulp.watch(config.themeDir + "/templates/*.twig", ['drushcr']); gulp.watch(config.themeDir + "/**/*.yml", ['drushcr']); gulp.watch(config.themeDir + "/**/*.theme", ['drushcr']); });
After that is all setup, if you have gulp globally installed, you will be able to run gulp
from the root of your project and the process will execute drushcr and begin watching your files. (Run npm install -g gulp
to install gulp globally)
This is an extremely simple example of what can be done with gulp and its many powerful plugins. We can easily create more tasks to help streamline our workflows with sass files or js/css minification or any other sort of preprocess that you want done to the code before it is passed to the browser. In our case we have a large number of projects with other workflows already in place, so our setup is as minimal as possible to avoid complications between projects.
Explore more posts in the Hark blog –>
Want to learn more about how Hark can help?